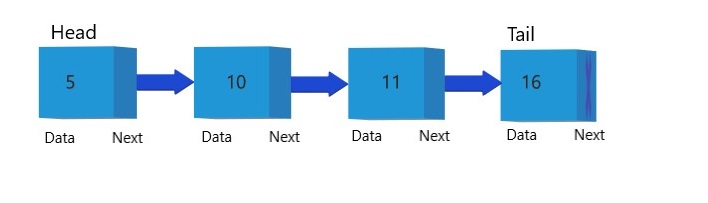
Linked List
Part 1 : Understanding the Basics
Topics Covered
- - What are Linked Lists?
- - Why Linked Lists?
- - What is a node?
- - Singly Linked Lists
- - Creating a Node Object
- - Creating a Linked List and appending multiple nodes.
Linked Lists
Linked List data-structures are a kind of sequence based containers to store your data and access a collection of data. List is one of the sequence based data-structures. In python, Lists come with a variety of features and methods to extract and manipulate the data. The python list can be automatically adjusted according to the need of the user.
Why Linked Lists?
The python list has some significant drawback as well:
- - Insertion and deletion are time consuming and tiresome, specially for huge collections of data
- - The length of the dynamic list is longer than it it requires
- - An expansion of the list requires the creation of another list, where original elements need to be copied and hence each time a list is created the program allocates a block of memory in which list is stored. Thus for large arrays, it is really difficult and not a good practice.
So to solve the aforementioned problems we have Linked List Data Structure. This provides an alternative to array-list data structures. It has efficient management and requires smaller memory allocations. It does eliminate the efficient way of accessing an element in constant time. Thus, it is not suited for all data storage problem.
Singly Linked List
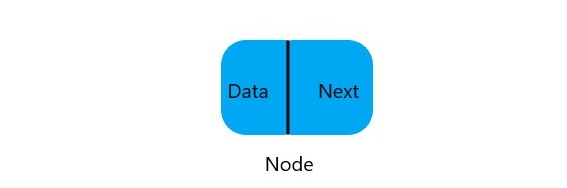
The Singly Linked List is a linear data structure in which traversal starts at the beginning and gradually progresses towards the end, one at a time. It is a collection of nodes that forms a linear sequence. The nodes store the reference to the next node and the element of the sequence.
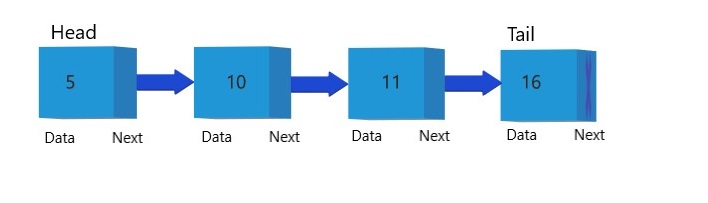
Most Nodes in the list have no names and are referenced by using the next field of the preceding node. The head pointer is referenced by a variable called head. A linked list can be empty, which indicates that the head reference is null.
Creating a Node Object
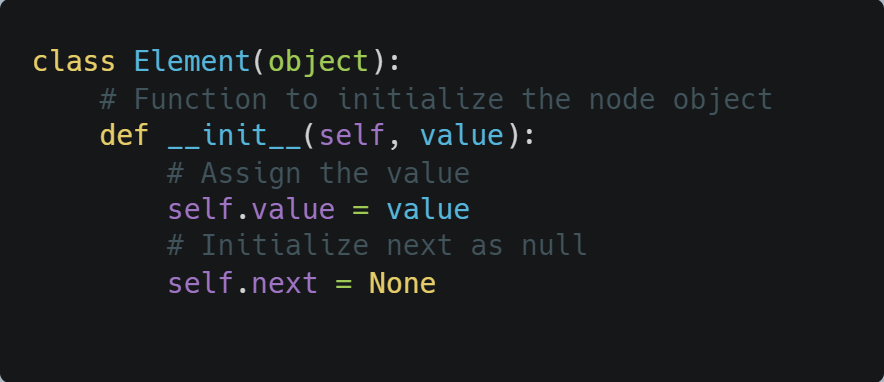
We create a class Element wherein we initialize the node object and assign a value and also initialize next as null value as well. Thus we have individual elements as nodes, we need to connect each other by referencing through the next value.
Creating a Linked List
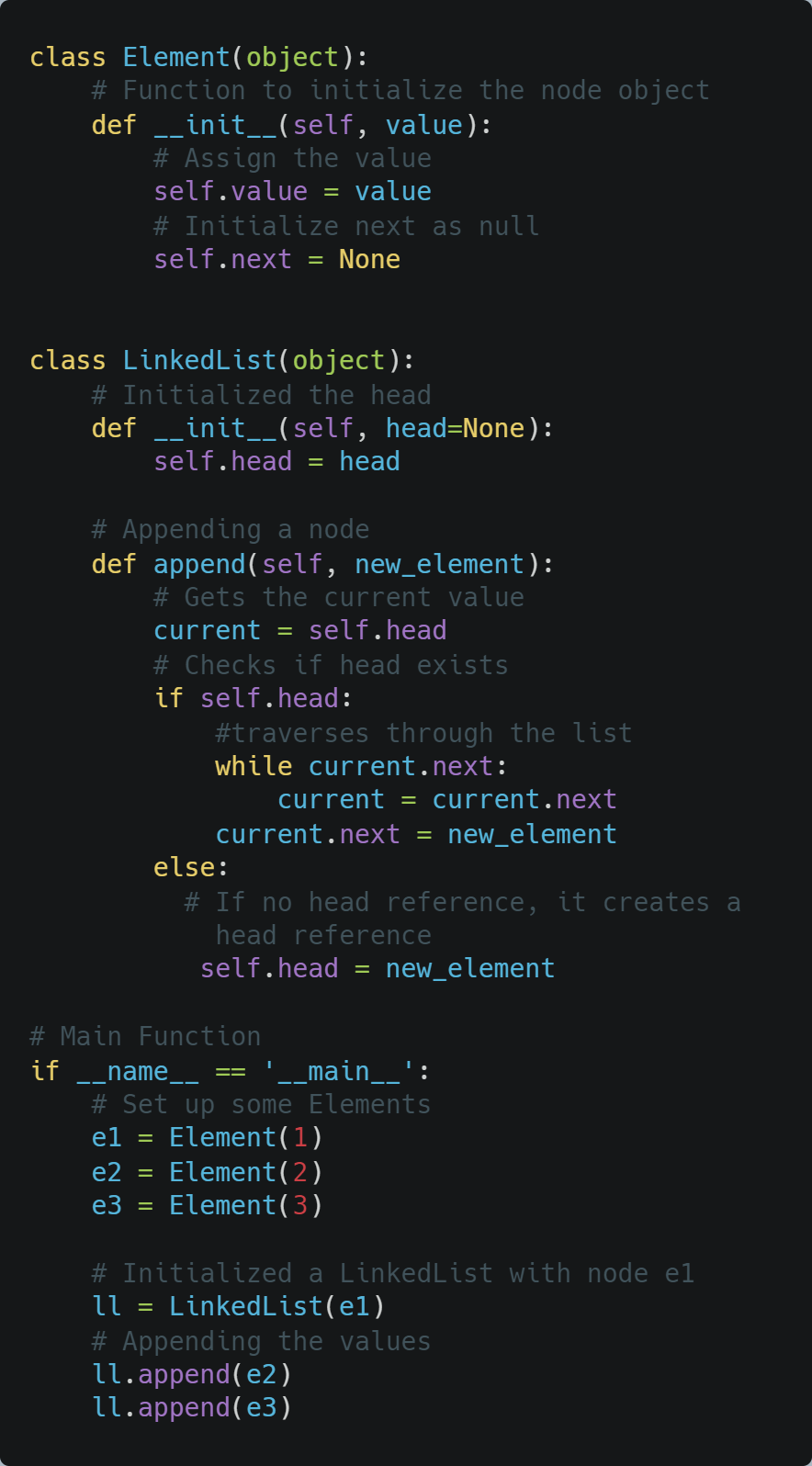
Creating a linked list involves creating multiple nodes and then appending a node at the beginning and referencing as head, rest of the nodes will be referenced using the next field of the preceding node.